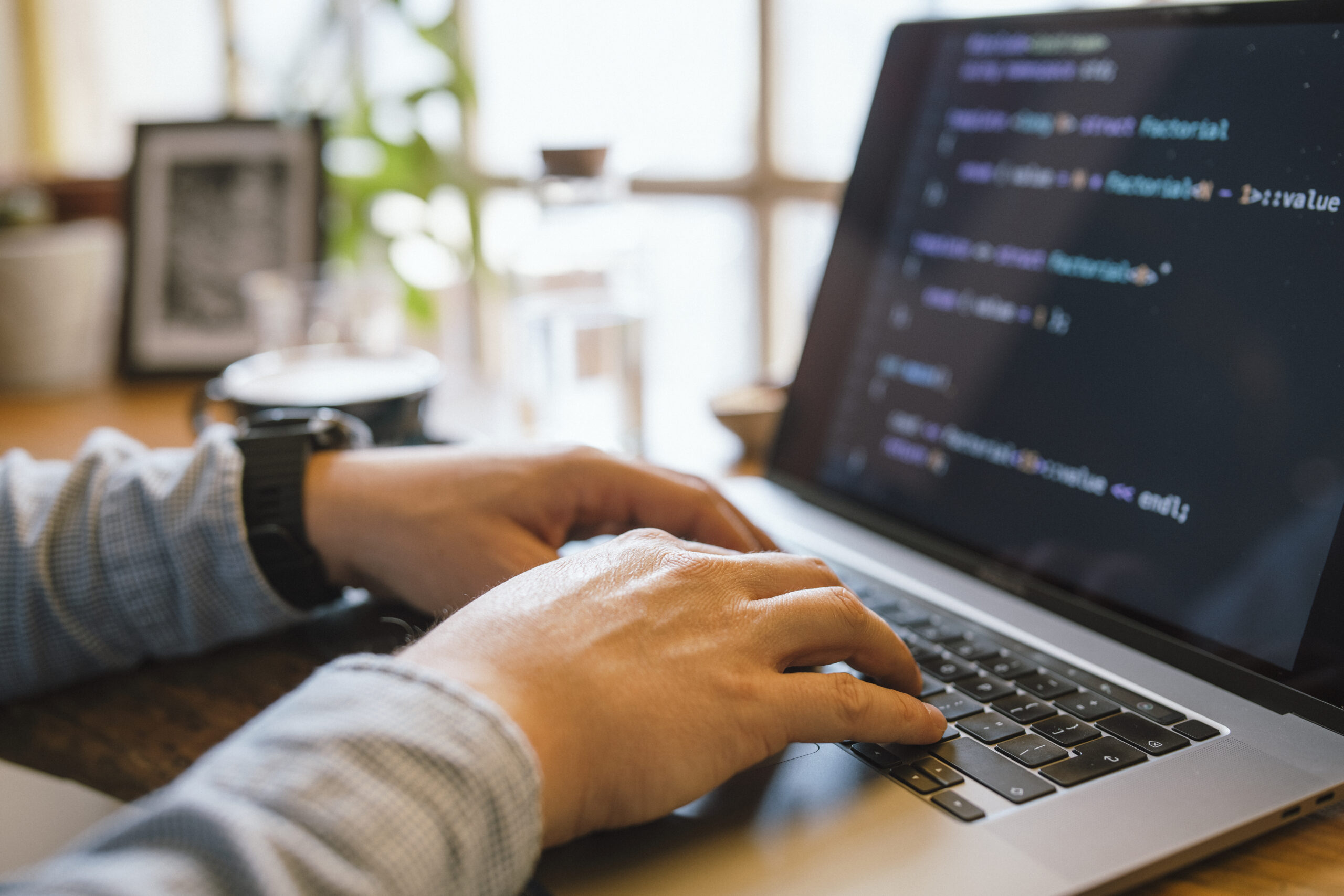
Debugging is Probably the most critical — but generally missed — capabilities inside a developer’s toolkit. It's not pretty much fixing broken code; it’s about knowing how and why factors go Completely wrong, and learning to Believe methodically to resolve problems effectively. No matter if you are a beginner or even a seasoned developer, sharpening your debugging capabilities can help save several hours of irritation and dramatically enhance your productiveness. Allow me to share various procedures to help developers stage up their debugging video game by me, Gustavo Woltmann.
Learn Your Applications
One of the quickest ways builders can elevate their debugging competencies is by mastering the instruments they use every day. While writing code is 1 A part of advancement, being aware of ways to connect with it proficiently all through execution is Similarly critical. Modern day progress environments come Geared up with impressive debugging capabilities — but numerous developers only scratch the surface of what these resources can do.
Just take, as an example, an Integrated Advancement Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, action by way of code line by line, and also modify code on the fly. When used appropriately, they let you notice accurately how your code behaves all through execution, which happens to be a must have for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-stop builders. They let you inspect the DOM, observe network requests, watch genuine-time general performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can turn discouraging UI troubles into manageable jobs.
For backend or procedure-stage developers, tools like GDB (GNU Debugger), Valgrind, or LLDB present deep Regulate around jogging procedures and memory administration. Finding out these instruments may have a steeper Understanding curve but pays off when debugging performance issues, memory leaks, or segmentation faults.
Further than your IDE or debugger, develop into comfortable with Model control devices like Git to know code background, locate the exact minute bugs were launched, and isolate problematic changes.
In the end, mastering your equipment suggests likely beyond default settings and shortcuts — it’s about building an intimate expertise in your enhancement natural environment to ensure when challenges occur, you’re not dropped in the dead of night. The higher you recognize your equipment, the greater time you may commit fixing the actual dilemma as an alternative to fumbling as a result of the method.
Reproduce the Problem
One of the more essential — and infrequently ignored — measures in productive debugging is reproducing the trouble. In advance of leaping into the code or generating guesses, developers want to create a consistent surroundings or scenario exactly where the bug reliably seems. Without reproducibility, repairing a bug will become a video game of probability, generally bringing about wasted time and fragile code improvements.
The first step in reproducing a dilemma is gathering as much context as you possibly can. Question queries like: What actions resulted in the issue? Which ecosystem was it in — development, staging, or generation? Are there any logs, screenshots, or mistake messages? The greater detail you've got, the less complicated it results in being to isolate the precise ailments less than which the bug occurs.
When you’ve gathered enough information and facts, try to recreate the situation in your neighborhood environment. This might mean inputting the identical knowledge, simulating comparable person interactions, or mimicking method states. If The difficulty appears intermittently, take into account producing automated assessments that replicate the edge cases or condition transitions involved. These assessments don't just support expose the issue but will also avert regressions Down the road.
At times, The difficulty might be environment-distinct — it'd occur only on specified functioning devices, browsers, or less than certain configurations. Applying equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms could be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t only a phase — it’s a mindset. It demands endurance, observation, in addition to a methodical method. But when you can regularly recreate the bug, you are by now midway to repairing it. Which has a reproducible scenario, You can utilize your debugging instruments additional effectively, test possible fixes securely, and talk far more Plainly with the workforce or people. It turns an summary criticism into a concrete problem — and that’s in which developers prosper.
Examine and Understand the Error Messages
Error messages tend to be the most worthy clues a developer has when a little something goes wrong. Instead of seeing them as frustrating interruptions, developers should learn to take care of mistake messages as immediate communications through the process. They usually tell you precisely what happened, where by it transpired, and from time to time even why it took place — if you understand how to interpret them.
Commence by studying the message carefully and in whole. Numerous developers, particularly when underneath time force, look at the very first line and instantly commence producing assumptions. But deeper from the error stack or logs may lie the legitimate root lead to. Don’t just copy and paste error messages into search engines like google and yahoo — browse and recognize them initial.
Crack the error down into pieces. Can it be a syntax mistake, a runtime exception, or even a logic error? Will it position to a specific file and line variety? What module or function activated it? These concerns can tutorial your investigation and stage you towards the responsible code.
It’s also helpful to grasp the terminology from the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java often follow predictable designs, and learning to recognize these can considerably increase your debugging method.
Some glitches are imprecise or generic, As well as in Those people instances, it’s very important to examine the context through which the error transpired. Check associated log entries, input values, and up to date changes while in the codebase.
Don’t ignore compiler or linter warnings either. These usually precede larger sized concerns and provide hints about prospective bugs.
Eventually, error messages will not be your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, serving to you pinpoint problems speedier, lower debugging time, and become a a lot more economical and self-assured developer.
Use Logging Sensibly
Logging is The most impressive equipment inside of a developer’s debugging toolkit. When made use of efficiently, it offers serious-time insights into how an application behaves, supporting you realize what’s happening beneath the hood without needing to pause execution or stage from the code line by line.
An excellent logging strategy begins with being aware of what to log and at what degree. Popular logging degrees include DEBUG, INFO, WARN, Mistake, and FATAL. Use DEBUG for in-depth diagnostic facts for the duration of improvement, Data for general functions (like thriving commence-ups), WARN for prospective issues that don’t break the appliance, ERROR for true complications, and Deadly when the technique can’t go on.
Stay clear of flooding your logs with abnormal or irrelevant facts. An excessive amount of logging can obscure important messages and slow down your system. Focus on vital occasions, condition adjustments, enter/output values, and demanding decision details inside your code.
Structure your log messages Obviously and consistently. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to track how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with no halting This system. They’re Particularly valuable in generation environments exactly where stepping by code isn’t attainable.
In addition, website use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with monitoring dashboards.
In the long run, sensible logging is about harmony and clarity. That has a effectively-believed-out logging approach, you could reduce the time it will take to identify issues, obtain further visibility into your purposes, and improve the Over-all maintainability and reliability of the code.
Imagine Just like a Detective
Debugging is not simply a technological job—it's a sort of investigation. To effectively recognize and resolve bugs, builders have to tactic the process just like a detective fixing a mystery. This way of thinking assists break down intricate difficulties into workable elements and comply with clues logically to uncover the basis bring about.
Get started by gathering evidence. Look at the signs of the situation: mistake messages, incorrect output, or general performance problems. Much like a detective surveys a crime scene, gather as much pertinent details as it is possible to devoid of leaping to conclusions. Use logs, examination conditions, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Request oneself: What could possibly be leading to this behavior? Have any modifications recently been made to the codebase? Has this difficulty happened in advance of beneath equivalent circumstances? The target would be to slim down options and determine prospective culprits.
Then, test your theories systematically. Try to recreate the condition in a very managed setting. Should you suspect a certain perform or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, inquire your code queries and let the results lead you closer to the reality.
Pay close interest to tiny specifics. Bugs generally cover from the minimum expected sites—just like a lacking semicolon, an off-by-just one error, or even a race issue. Be comprehensive and individual, resisting the urge to patch the issue without the need of entirely knowing it. Temporary fixes may cover the real challenge, only for it to resurface later.
Last of all, hold notes on Everything you tried using and uncovered. Equally as detectives log their investigations, documenting your debugging process can help save time for future troubles and assistance Other individuals recognize your reasoning.
By wondering similar to a detective, developers can sharpen their analytical capabilities, technique challenges methodically, and come to be more effective at uncovering concealed issues in intricate methods.
Publish Assessments
Composing exams is one of the most effective ways to transform your debugging skills and In general improvement efficiency. Tests not only help capture bugs early but also function a security Internet that provides you assurance when earning variations towards your codebase. A very well-tested application is easier to debug since it permits you to pinpoint just wherever and when a challenge takes place.
Get started with device assessments, which deal with unique functions or modules. These tiny, isolated tests can quickly reveal no matter if a certain piece of logic is Functioning as anticipated. When a check fails, you instantly know the place to glimpse, noticeably cutting down enough time put in debugging. Unit tests are especially practical for catching regression bugs—challenges that reappear immediately after previously being preset.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These help make sure various aspects of your application operate together smoothly. They’re specifically valuable for catching bugs that come about in elaborate systems with many elements or solutions interacting. If anything breaks, your checks can inform you which A part of the pipeline unsuccessful and below what conditions.
Producing tests also forces you to definitely Feel critically regarding your code. To test a function adequately, you would like to understand its inputs, predicted outputs, and edge instances. This volume of knowing Normally qualified prospects to raised code construction and fewer bugs.
When debugging a difficulty, writing a failing test that reproduces the bug might be a powerful initial step. Once the take a look at fails continually, you'll be able to deal with fixing the bug and look at your exam move when The difficulty is resolved. This strategy makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the irritating guessing video game into a structured and predictable process—encouraging you catch extra bugs, speedier and more reliably.
Consider Breaks
When debugging a tough situation, it’s quick to become immersed in the trouble—observing your monitor for several hours, trying Remedy soon after Option. But The most underrated debugging tools is simply stepping away. Using breaks will help you reset your head, lower irritation, and infrequently see The difficulty from a new point of view.
When you're far too near the code for much too long, cognitive exhaustion sets in. You might start overlooking obvious errors or misreading code that you wrote just several hours before. During this point out, your Mind gets considerably less productive at dilemma-fixing. A short walk, a espresso crack, or maybe switching to a distinct process for 10–15 minutes can refresh your aim. Lots of builders report locating the root of a problem once they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also enable stop burnout, Specifically through longer debugging classes. Sitting in front of a display, mentally caught, is not simply unproductive but will also draining. Stepping absent enables you to return with renewed Vitality and also a clearer way of thinking. You might suddenly discover a lacking semicolon, a logic flaw, or simply a misplaced variable that eluded you right before.
In case you’re stuck, a great rule of thumb would be to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It could really feel counterintuitive, Primarily beneath limited deadlines, nonetheless it essentially results in a lot quicker and more effective debugging In the long term.
In brief, having breaks isn't an indication of weak spot—it’s a smart tactic. It gives your brain House to breathe, enhances your perspective, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Understand From Each Bug
Each and every bug you come upon is a lot more than simply a temporary setback—It can be a possibility to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or even a deep architectural difficulty, every one can instruct you a little something beneficial for those who make time to replicate and review what went Mistaken.
Start out by inquiring you a few crucial queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved practices like device tests, code reviews, or logging? The responses often expose blind places in the workflow or understanding and assist you to Develop much better coding habits moving ahead.
Documenting bugs will also be a wonderful pattern. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll start to see styles—recurring difficulties or widespread problems—which you can proactively stay away from.
In group environments, sharing what you've learned from the bug using your peers can be Primarily highly effective. Whether it’s through a Slack information, a brief generate-up, or A fast know-how-sharing session, supporting Other people steer clear of the very same problem boosts group performance and cultivates a more robust Studying society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial parts of your progress journey. In the end, a lot of the ideal builders will not be those who publish best code, but those who repeatedly discover from their faults.
In the end, Every single bug you fix adds a completely new layer in your skill set. So following time you squash a bug, have a moment to mirror—you’ll occur away a smarter, far more able developer due to it.
Summary
Improving upon your debugging expertise can take time, apply, and endurance — though the payoff is huge. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to become far better at That which you do.